Here is a tutorial on how to show items IDs on the display and edit forms.
We are able to display the list item IDs when viewing the list, but the IDs are not present when we open or edit the items.
To add IDs to the display and edit forms we need to first know their URLs
Default display form name: DispForm.aspx
- Full URL example (http://localhost/Lists/Sales/DispForm.aspx)
Default edit form name: EditForm.aspx
- Full URL example (http://localhost/Lists/Sales/EditForm.aspx)
(if you are using custom forms with different names you can use SP Designer to check the default form names)
To edit these forms from the browser like they were a page we can add the text ?toolpaneview=2 to the end of the URL.
This will make the page edit tools available to you.
Add a HTML Form Web Part to the page, now we can add our own custom code to the page.
This solution requires JQuery so you have 2 choices on how to reference it.
- If you are in an environment where you have unrestricted access to the internet you can reference JQuery through the google APIs.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script>
- If you are in an environment where internet access is restricted you can download a copy of JQuery and upload it to a SharePoint library then reference it.
<script src="http://localhost/Shared%20Documents/jquery-1.10.2.min.js"></script>
Once you figure out which JQuery you are going to go with copy and paste the code below into the Source.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script>
<script type="text/javascript">
$(function() {
// Get ID
var id = getQueryString()["ID"];
// Get the main form table
var maintable = $('table.ms-formtable');
// Now add a row to the start of the table
maintable.prepend("<tr><td class='ms-formlabel'><h3 class='ms-standardheader'>ID</h3></td>" +
"<td class='ms-formbody'>" + id + " </td></tr>");
})
function getQueryString() {
var assoc = new Array();
var queryString = unescape(location.search.substring(1));
var keyValues = queryString.split('&');
for (var i in keyValues) {
var key = keyValues[i].split('=');
assoc[key[0]] = key[1];
}
return assoc;
}
</script>
|
You should then see the ID field appear on all the forms.
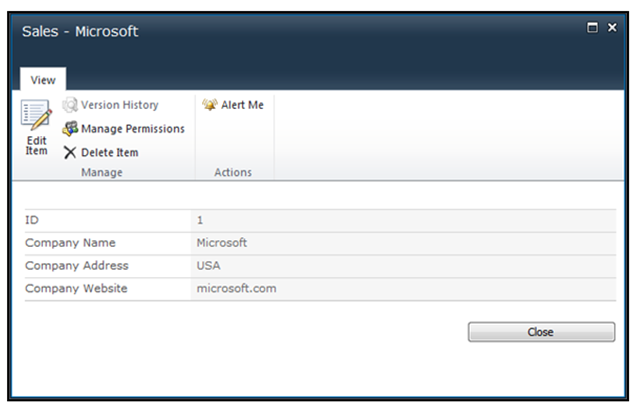
This process will need to be done on both forms (edit and display). On the edit form the ID will appear but not be editable.